If you are a technical professional or a developer working with SQL Server, then you are most likely familiar with T-SQL (Transact-SQL). T-SQL is the primary language used for querying and managing data in SQL Server. It is a powerful and versatile language that allows you to perform a wide range of tasks, from creating stored procedures and SQL functions to implementing transactions and writing complex SQL queries. In this article, we will provide a comprehensive guide to T-SQL, covering its key features, syntax, and best practices. Whether you are new to T-SQL or looking to enhance your skills, this guide will serve as a valuable resource for mastering this essential language.
What is T-SQL?
T-SQL (Transact-SQL) is an extension to the SQL (Structured Query Language) used for managing data in relational databases. It was developed by Microsoft and is the standard language for managing data in SQL Server. T-SQL includes additional programming constructs and features, making it a powerful language for creating complex database applications.
Key Features of T-SQL
T-SQL offers a wide range of features and capabilities that make it one of the most popular languages for working with SQL Server. Some of its key features include:
1. Stored Procedures: T-SQL allows you to create stored procedures, which are pre-compiled blocks of SQL statements that can be executed whenever needed. Stored procedures offer many advantages, such as improved performance, code reusability, and enhanced security.
2. SQL Functions: In addition to stored procedures, T-SQL also supports the creation of user-defined functions. These functions can be used to perform calculations, manipulate data, or return results based on specific criteria.
3. Cursors: Cursors in T-SQL are used to loop through a result set and perform operations on each row of data. This allows for greater control and flexibility when working with data in SQL Server.
4. Transactions: T-SQL supports transaction processing, which enables you to group related database operations into a single unit. This ensures data consistency and helps maintain the integrity of the database.
5. SQL Queries: T-SQL is primarily used for writing SQL queries to retrieve and manipulate data in databases. With its powerful syntax and functions, T-SQL allows for complex and efficient retrieval of data.
Syntax and Basic Structure of T-SQL
T-SQL follows a similar syntax and structure to SQL but includes additional constructs to support its extended features. The basic structure of a T-SQL statement is as follows:
COMMAND [additional keywords] [databaseName].[schemaName].[objectName] [additional clauses]
The COMMAND can be any T-SQL statement, such as SELECT, INSERT, UPDATE, or DELETE. The additional keywords can include conditions, operators, and functions. The database name, schema name, and object name specify the location of the data being operated on. Additional clauses can be included at the end, such as WHERE, GROUP BY, ORDER BY, etc.
Let’s take a look at some examples of T-SQL statements:
Select all rows from a table called ’employees’ in the ‘company’ database:
SELECT * FROM company.dbo.employees
Update the salary of an employee with ID 101 to $50,000:
UPDATE company.dbo.employees SET salary = 50000 WHERE id = 101
Create a stored procedure to retrieve all employees hired after a specific date:
CREATE PROCEDURE GetEmployeesByDate
@hired_date DATE
AS
BEGIN
SELECT * FROM company.dbo.employees WHERE hire_date > @hired_date
END
These are just a few examples of how T-SQL can be used to interact with a SQL Server database. The possibilities are endless, and the syntax can be adapted to fit a wide range of scenarios.
Best Practices for Writing T-SQL code
To ensure efficient and effective use of T-SQL, it is essential to follow some best practices while writing code. Some of these best practices include:
1. Use Proper Indentation: Proper indentation improves code readability, making it easier to identify the structure of the code and find any potential errors.
2. Use Appropriate Naming Conventions: Choose descriptive and meaningful names for database objects, such as tables, columns, stored procedures, and functions. This makes it easier to understand the purpose of each object.
3. Avoid Using SELECT *: In most cases, it is recommended to specifically select the columns needed rather than using SELECT * to retrieve all columns. This can improve performance and reduce the amount of unnecessary data being retrieved.
4. Use SET NOCOUNT ON: Setting NOCOUNT ON at the beginning of a stored procedure or function can improve performance by eliminating the message indicating the number of rows affected by the query.
5. Handle Errors and Exceptions: Proper error handling is crucial in T-SQL. Use TRY-CATCH blocks to handle potential errors, and use appropriate error messages for better troubleshooting.
6. Use Comments: Adding comments to your code can be helpful for future reference and also improve collaboration with other developers working on the same project.
Common Mistakes to Avoid:
While working with T-SQL, developers may make some common mistakes that can lead to errors or performance issues. These mistakes include:
1. Not using proper data types: Choosing the right data type for columns is crucial in T-SQL. Using the wrong data type can lead to unnecessary storage and performance issues.
2. Not considering data conversions: Converting data from one type to another can lead to errors and performance problems if not done correctly. It is essential to be aware of the data types being used and how they may be converted when performing operations.
3. Using too many cursors: Cursors should only be used when necessary as they can significantly impact performance. It is recommended to find alternative approaches or limit the use of cursors whenever possible.
4. Not using proper error handling: Neglecting proper error handling can make it difficult to troubleshoot issues and lead to unexpected errors in code. Always use TRY-CATCH blocks and appropriate error messages.
Alternative Approaches:
In some cases, there may be alternative approaches that can improve the efficiency and readability of T-SQL code. These include:
1. Using table variable instead of a temporary table: Table variables can provide better performance compared to temporary tables, especially for smaller datasets.
2. Avoiding the use of scalar functions in SELECT statements: Scalar functions called in SELECT statements can degrade query performance. Consider using derived tables or CTEs instead.
3. Using SET based operations instead of RBAR (Row-By-Agonizing-Row): RBAR operations can significantly impact performance. Using SET operations can improve the efficiency of T-SQL code and should be considered whenever possible.
By following best practices and avoiding common mistakes, developers can ensure that T-SQL code is optimized for performance and readability, making it easier to maintain and troubleshoot in the long run.
Conclusion
In this guide, we have covered the key concepts and features of T-SQL, along with its syntax and best practices for writing code. T-SQL is a versatile language that offers numerous capabilities for managing and manipulating data in SQL Server. By following the best practices and experimenting with the various features of T-SQL, developers can become proficient in using this language to its full potential and enhance their SQL Server skills.
References
Free Microsoft course: Get started with Transact-SQL programming
Reference documentation by Microsoft: click here
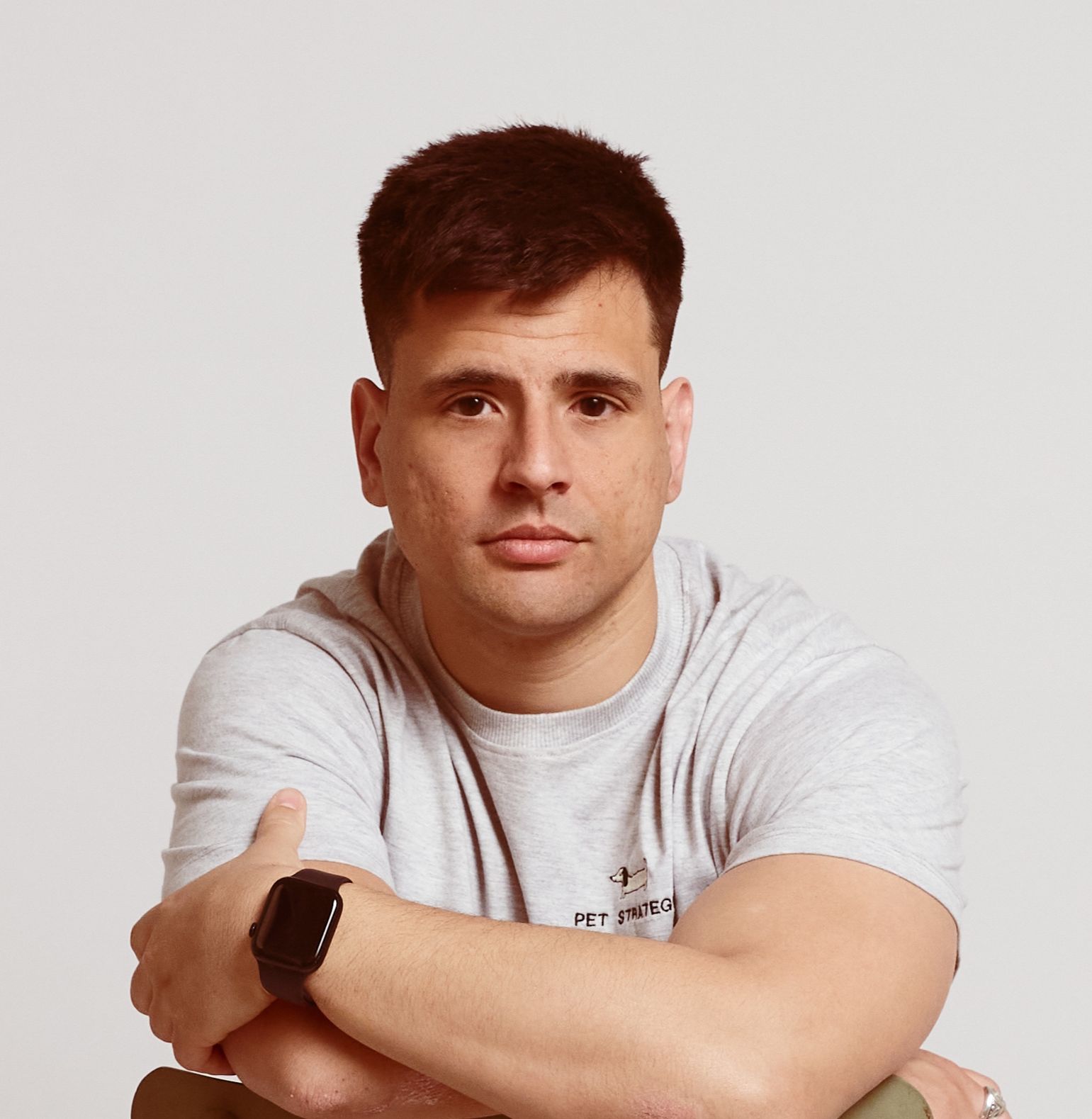
Specialized in scalability, automation, and security. Experienced in Kubernetes, AWS, and Azure, he ensures performance and reliability. Pursuing a Ph.D. in TCP congestion control, he holds an M.S. in Information Security and a B.S. in Telematics Engineering.